React Native EU 2022 was held couple of weeks ago and it’s a conference which focuses exclusively on React Native but consists also on general topics which are universal in software development while applied to RN context. This year the online event provided great talks and especially there were many presentations about apps performance improvements, achieving better code and identifying bugs. Here are my notes from the talks I found interesting. All of the talks are available in conference stream on Youtube.
Better performance and quality code from RN EU 2022
This year the React Native EU talks had among other presentations two common topics: performance and code quality. Important aspects of software development which are often dismissed until problems arise so it was refreshing to see it talked so much about.
Here are my notes from the talks I found most interesting. Also the “Can’t touch this” talk about accessibility was a good reminder that not everyone use their mobile devices by hand.
How we made our app 80% faster, a data structure story
Marin Godechot gave an interesting talk of React Native apps performance improvements and one of the crucial tools for achieving that.
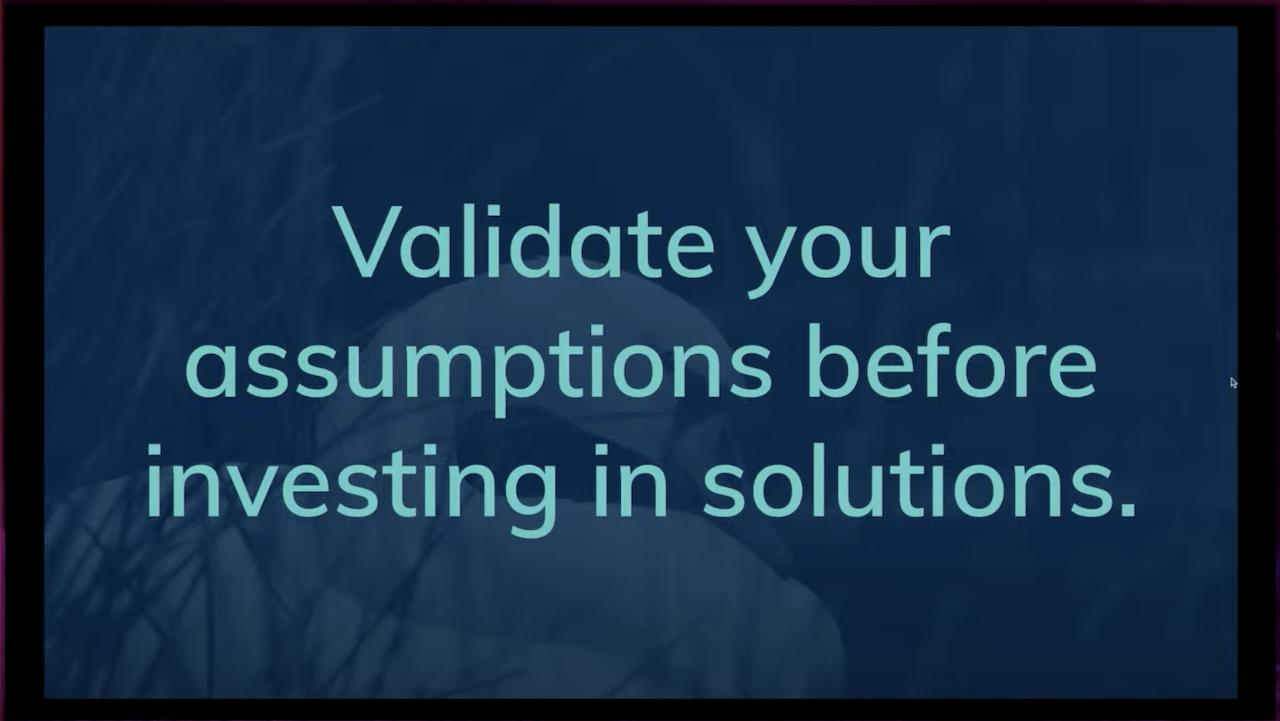
The learnings of the talk which went through trying different approaches to fix the performance problem was that:
- Validate your assumption before investing in solutions
- Performance improvements without tooling is a guessing game
- Slow Redux selectors are bad
- Data structures and complexity matters
The breakthrough for finding the problem was after actually measuring the performance with Datadog Real User Monitoring (RUM) and better understanding of the bottlenecks. What you can’t measure, you can’t improve.
The issue wasn’t with useless rerenders, lists and navigation stack but with the datamodels but not the one you would guess. Although the persisted JSON stringified state with Redux was transferred between the JS side and the Native (JS <-> json <-> Bridge <-> json <-> Native) it wasn’t the issue.
The big reveal was that when they instrumented Redux’s selectors, reducers and sagas to monitoring tool they found that as the user permissions were handled by using the Attribute Based Access Control model the data in JSON format had grown over the years from 15 permissions per user to 10000 permissions per user which caused problems with Redux selectors. The fix was relatively simple: change array to object -> {agengy: [agency:caregivers:manage]}
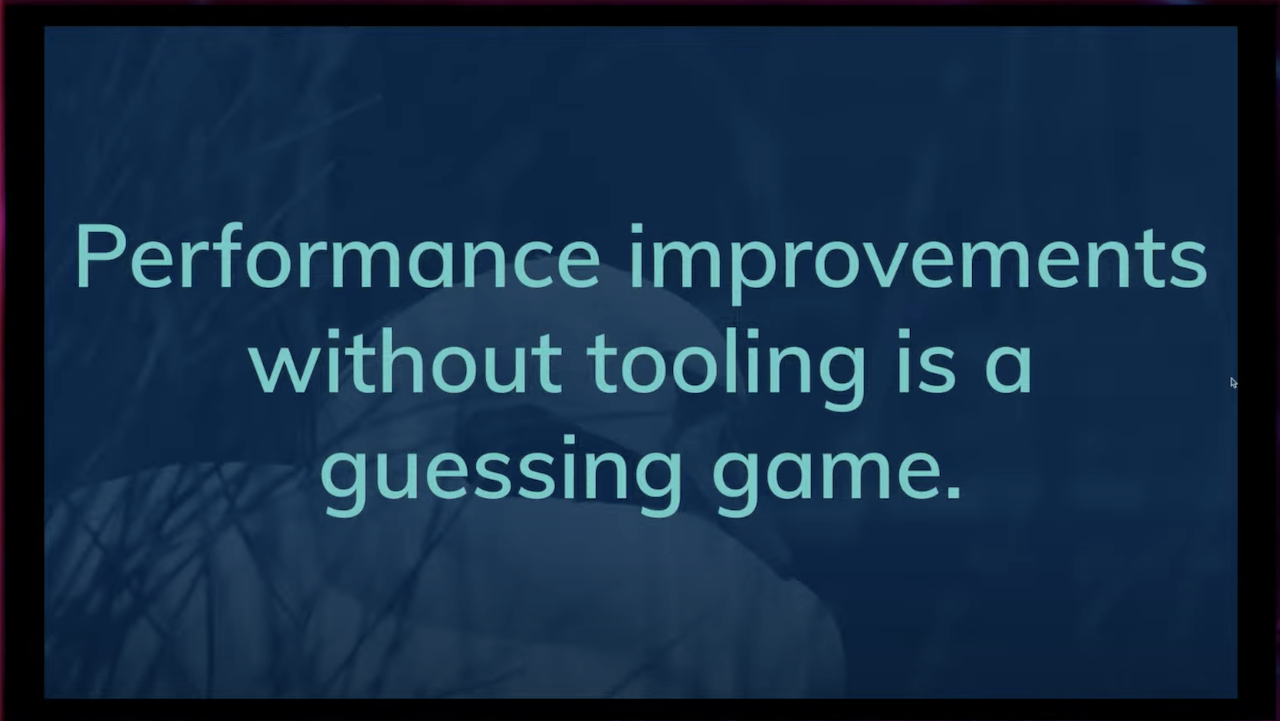
You can go EVERYWHERE but I can go FAST – holistic case study on performance
Jakub Binda made a clever comparison in his talk that apps are like cars and same modifications apply to fast cars and fast applications.
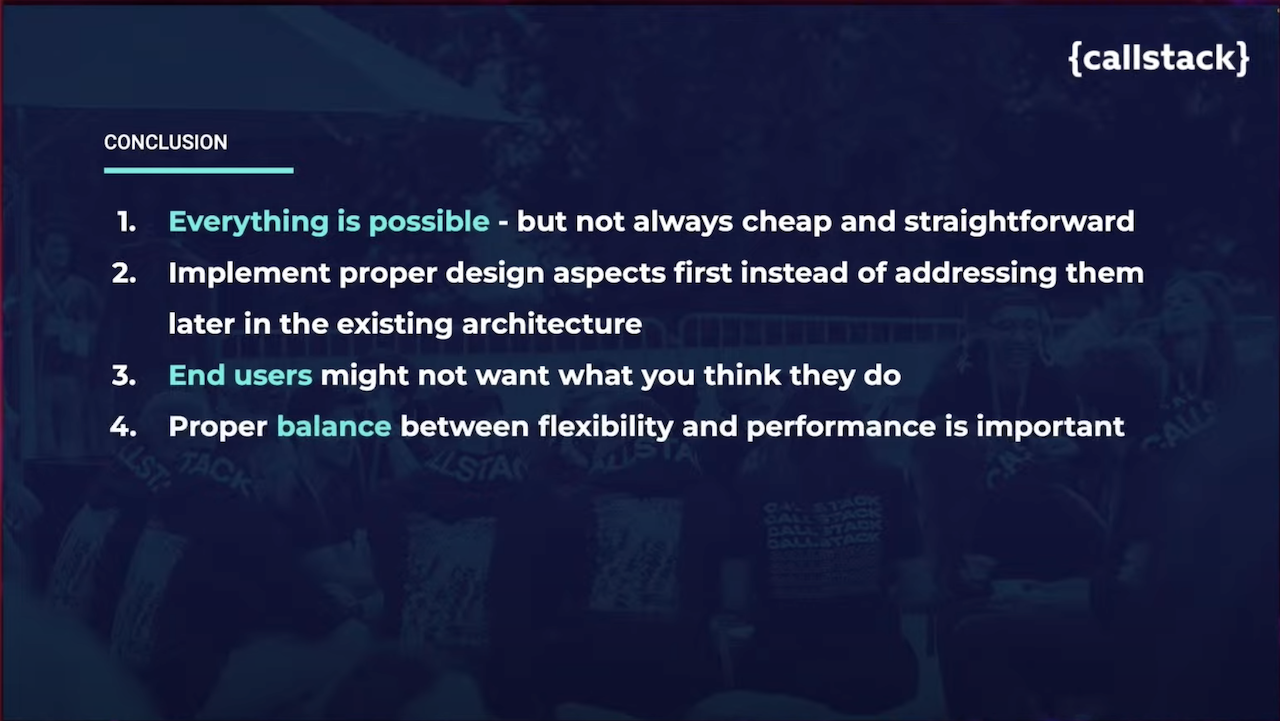
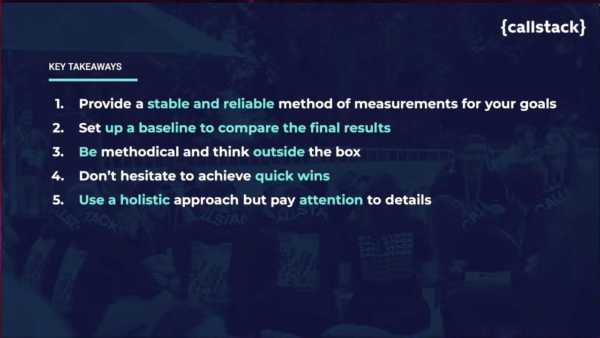
The talk starts slow but gets to speed nicely with good overview how to create faster apps. Some of the points were:
- Reduce weight:
- Remove dead / legacy code
- Avoid bundling heavy JSON files (e.g. translations)
- Keep node_modules clean
- Keep component structure “simple”
- More complex structure leads to longer render time and more resources consumption and more re-renders
- Shimmers are complex and heavy (improve UX by making an impression that content appers faster than it actually does)
- Using hooks:
- Might lead to unexpected re-renders when: their value change, dependency array is not set correctly
- Lead to increased resources comsumption if: their logic is complex, the consumer of the results is hidden behind the flag
- Tooling:
- Flipper debug tool: flame charts & performance monitoring tooling
Reducing bugs in a React codebase
Darshita Chaturvedi’s hands-on and thoroughly explained talk for identifying bugs in React codebase was insightful.
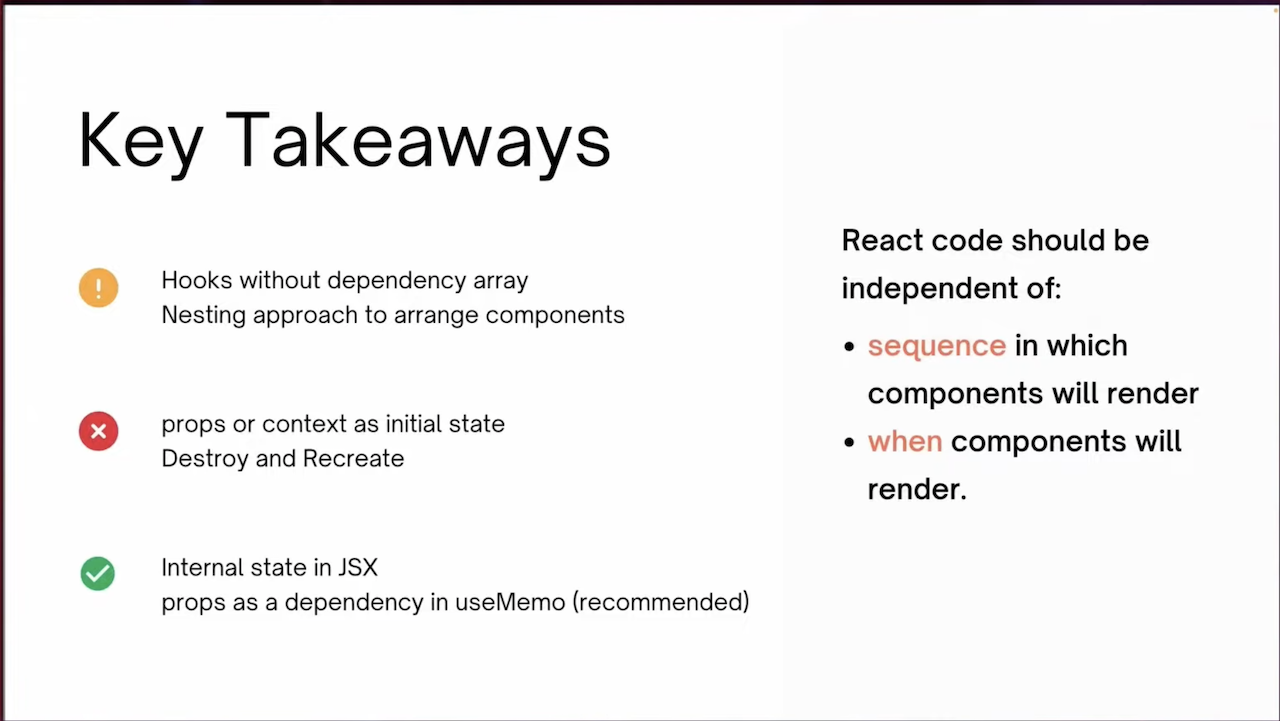
Getting Better All the Time: How to Escape Bad Code
Josh Justice had a great presentation with hands-on example of an application with needed fixes and features and how to apply them with refactoring. And what is TDD by recreating the application.
The slides are available with rest of the TDD sequence and pointers to more resources on TDD in RN.
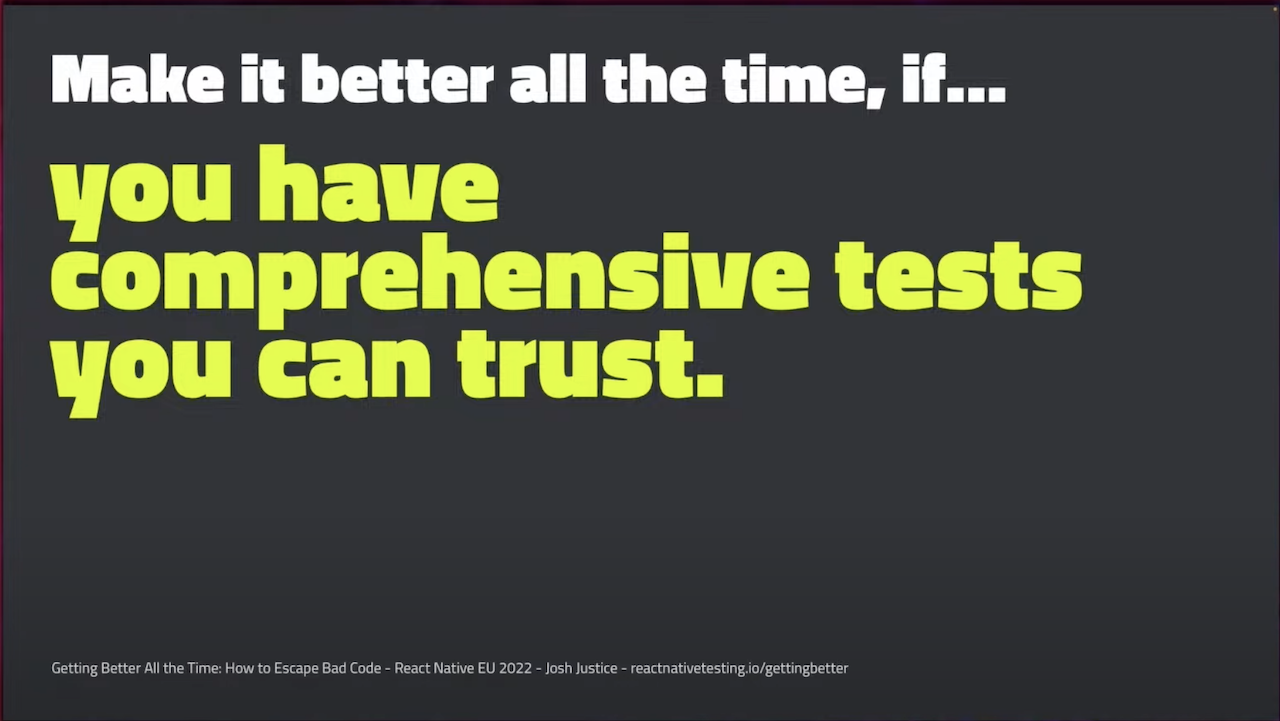
The key point of the talk was about what do you do about bad code? Do you work around bad code or rewrite bad code? The answer is refactoring: small changes that improve the arrangements of the code without changing its functionality. And comprehensive tests will save you with refactoring. The value is that you’re making the improvements that pay off right away and code is shippable after each refactoring. But how do you get there? By using Test-Driven Development (TDD)
“TDD is too much work”
Getting Better All the Time: How to Escape Bad Code
Living with bad code forever is also a lot of work
“Make code better all the time (with refactoring and better test coverage)”
Visual Regression Testing in React Native
Rob Walker talked about visual regression testing and about using React Native OWL. Slides available.
- Visual regression testing for React Native
- CLI to build and run
- Jest matcher
- Interaction API
- Report generator
- Inspired by Detox
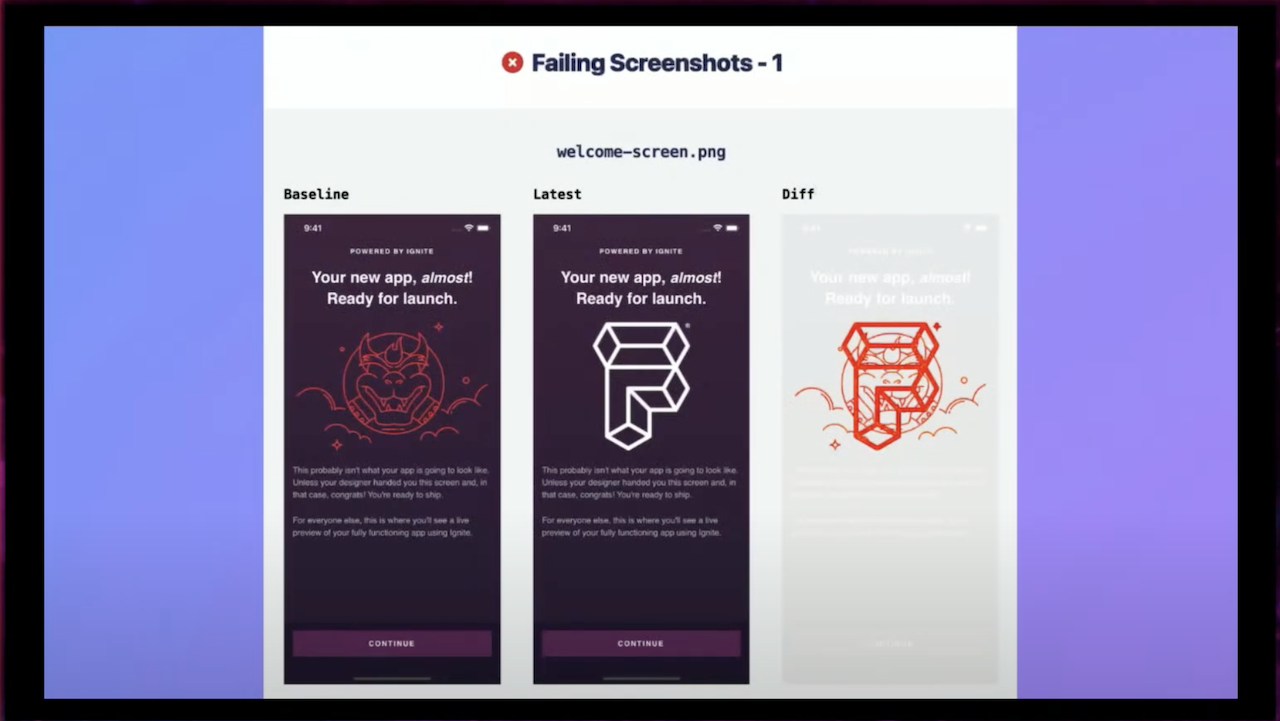
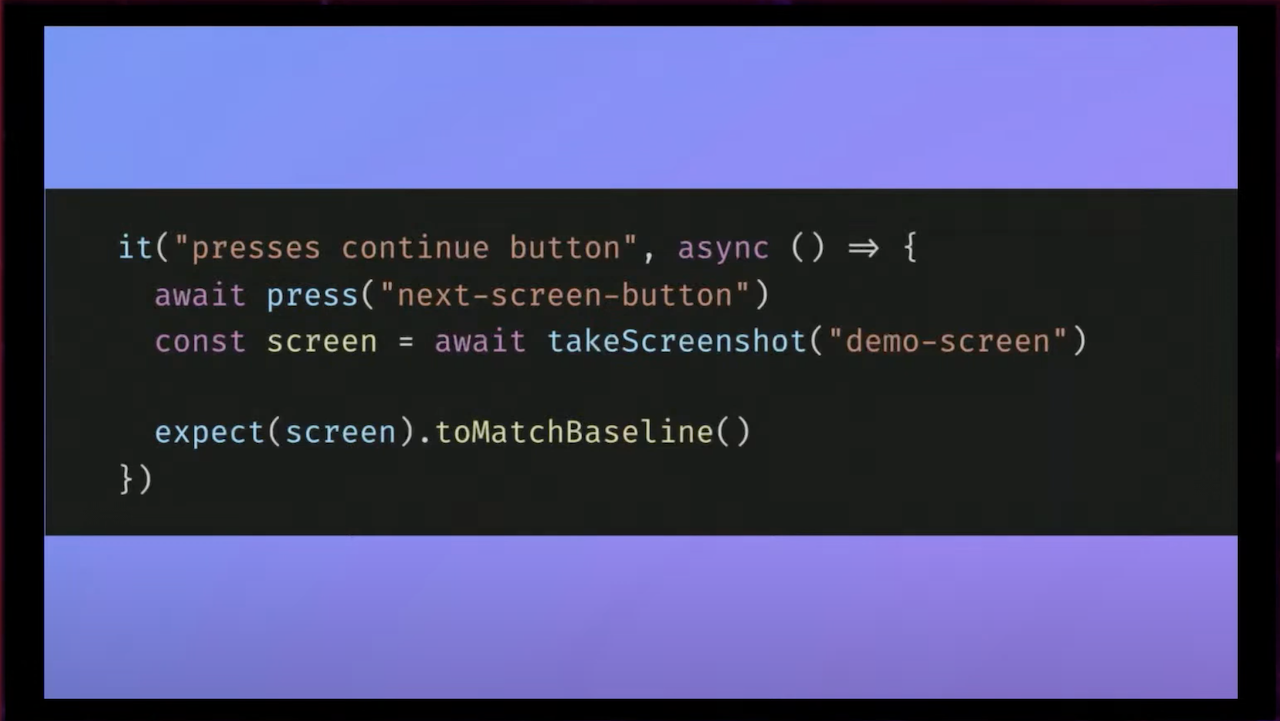
Different test types:
- Manual testing pros: great for exploratory testing, very flexible, can be outsourced
- Manual testing cons: easy to miss things, time consuming, hard to catch small changes
- Jest snapshot tests pros: fast to implement, fast to run, runs on CI
- Jest snapshot tests cons: only tests in isolation, does not test flow, only comparing JSX
- Visual regression tests pros: tests entire UI, checks multi-step flows, runs on CI
- Visual regression tests cons: can be difficult to setup, slower to run, potentially flaky
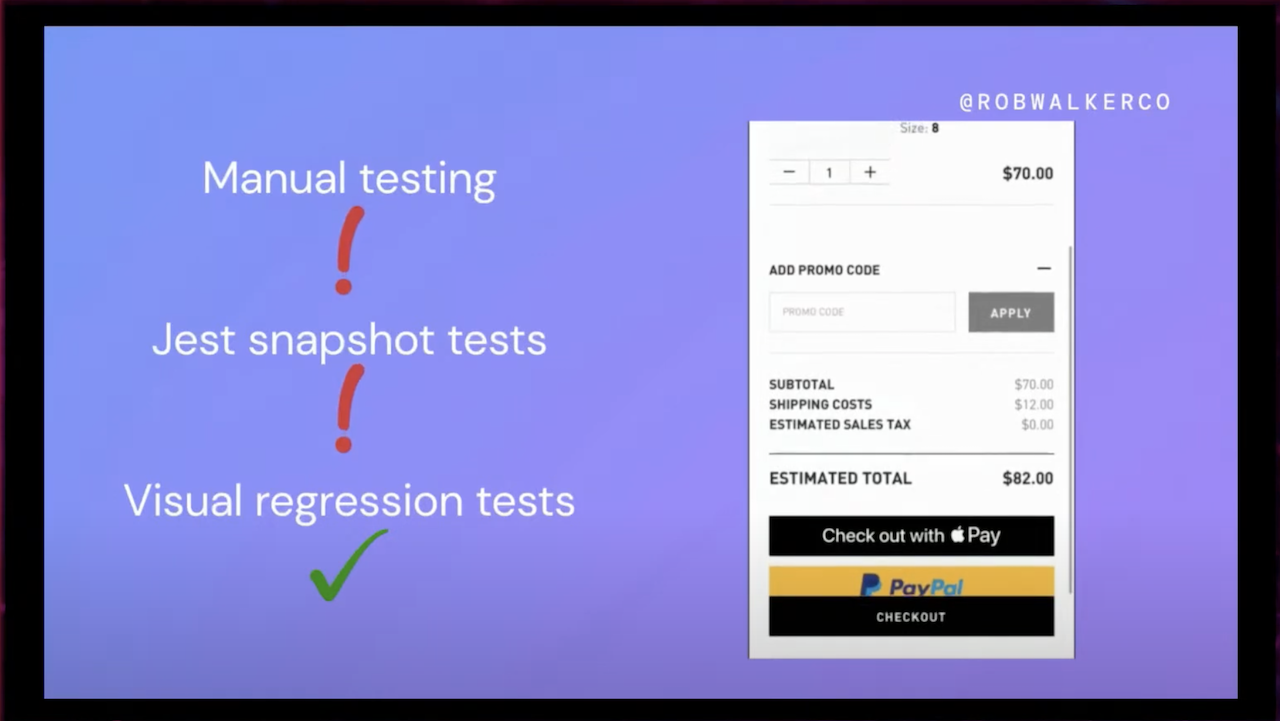
Can’t touch this – different ways users interact with their mobile devices
Eevis talked about accessibility and what does this mean for a developer. The slides give a good overview to the topic.
- Methods talked about
- Screen reader: trouble seeing or understanding screen, speech or braille feedback, voiceover, talkback
- Physical keyboard: i.a. tremors
- Switch devices: movement limiting disabilites
- Voice recognition
- Zooming / screen magnifying: low vision
- 4 easy to start with tips
- Use visible focus styles
- Annotate headings (accessibilityRole)
- Add name, role and state for custom elements
- Respect reduced motion
- Key takeaways
- Users use mobile devices differently
- Test with different input methods
- Educate yourself
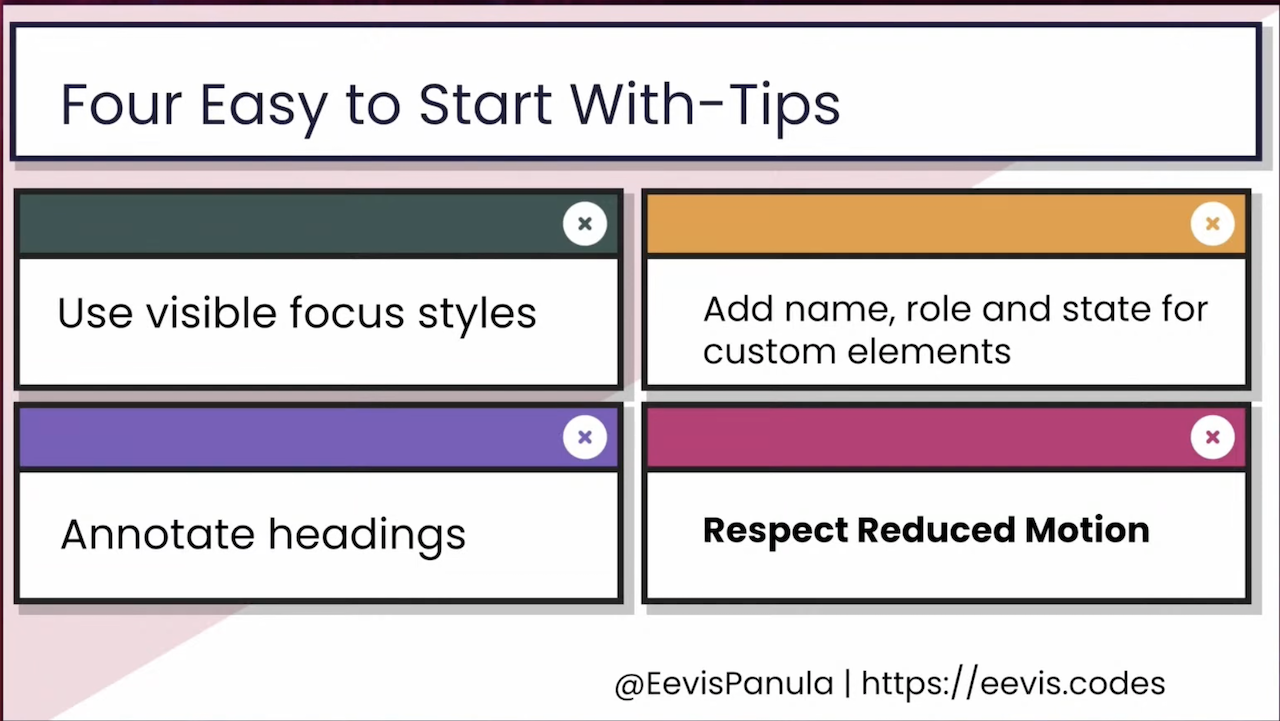
Performance issues – the usual suspects
Alexande Moureaux hands-on presentation of how to use Flipper React DevTools profiler and Flamegraph and Android performance profiler to debug Android application performance issues. E.g. why the app has below 60 fps? Concentrates on debugging Android but similar approaches work also for iOS.
First make your measures deterministic, average your measures over several iterations, keep the same conditions for every measure and automate the behavior you want to test.
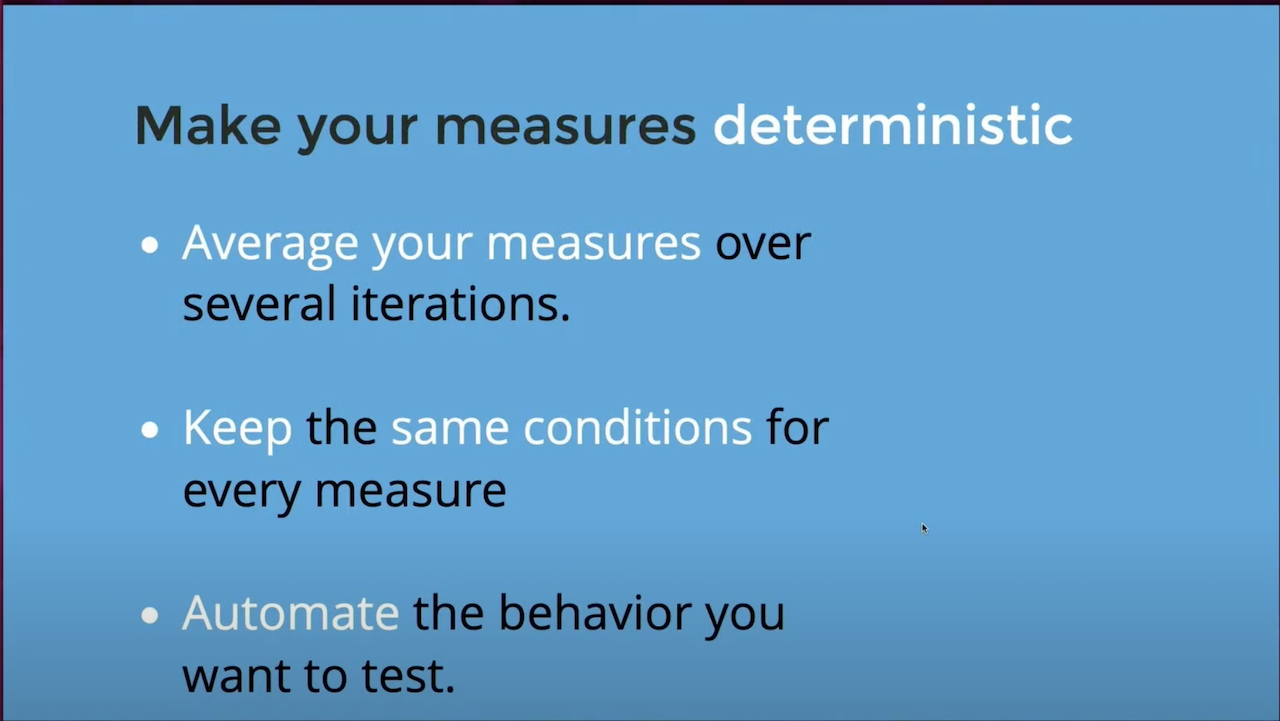
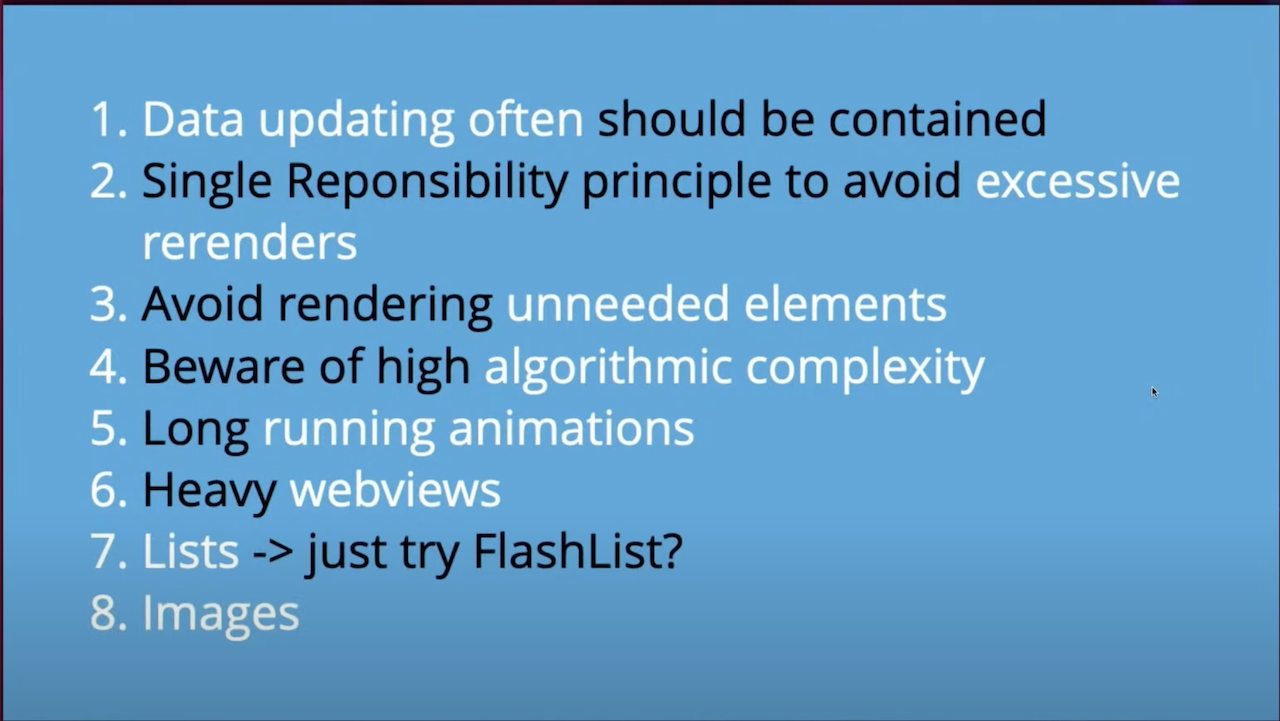
The presentation showed how to use Flipper React DevTools profiler Flamegraph to find which component is slow and causes e.g. rerendering. Then refactoring the view by moving and the updating component which caused rerendering. And using @perf-profiler/web-reporter to visualize the measures from JSON files.
You can record the trace Hermes profiler and open it on Google Chrome:
- bundleInDebug: true
- Start profiler in simulator, trace is saved in device
npx react-native profile-hermes
pulls the trace and converts it for usable format- Findings: filtering tweets list by parsing weekday from tweet was slow (high complexity), replace it with Date.getDay from tweet.createdAt
Connect the app to Android Studio for profiling: finding long running animation (background skeleton content “grey boxes”)
How to actually improve the performance of a RN App?
Michal Chudziak presented how to use Define, Measure, Analyze, Improve, Control (DMAIC) pattern to improve performance of a React Native application.
- Define where we want to go, listen to customers
- Make it measurable
- Analyze common user paths
- Choose priorities
Define your goals:
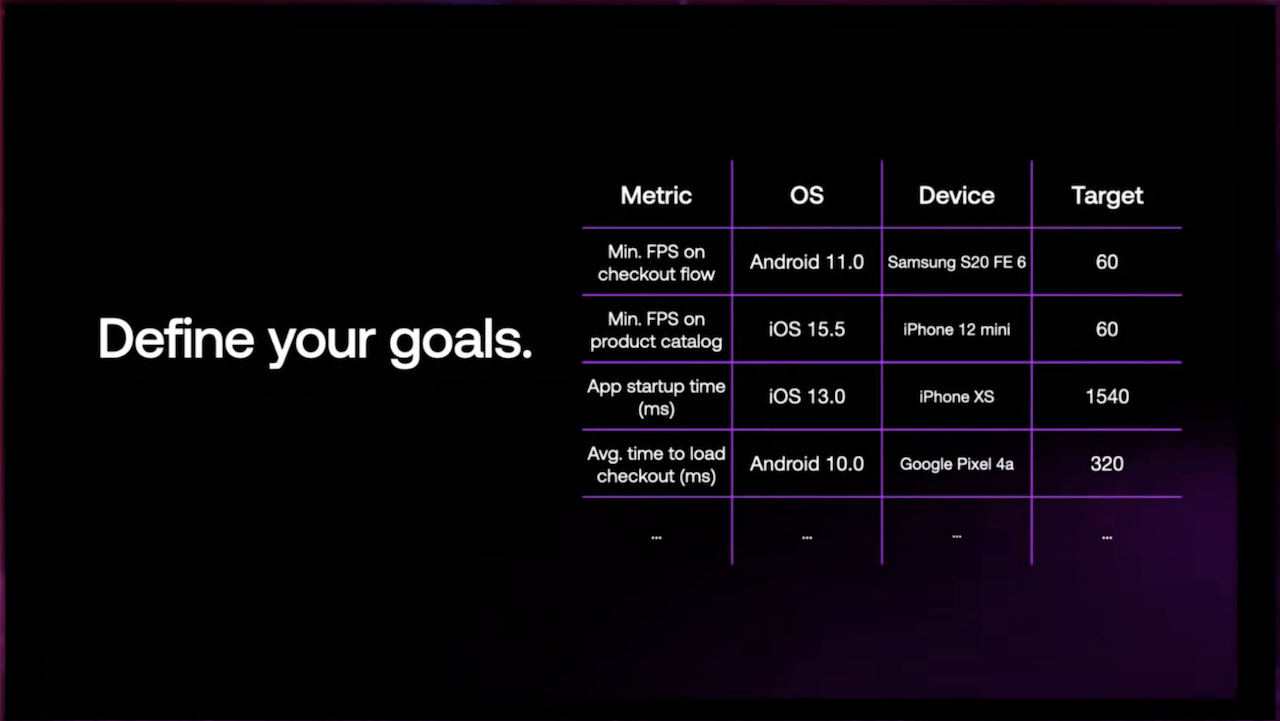
Measure: where are we?
- React Profiler API
- react-native-performance
- Native IDEs
- Screen capture
- Flipper & perf monitor
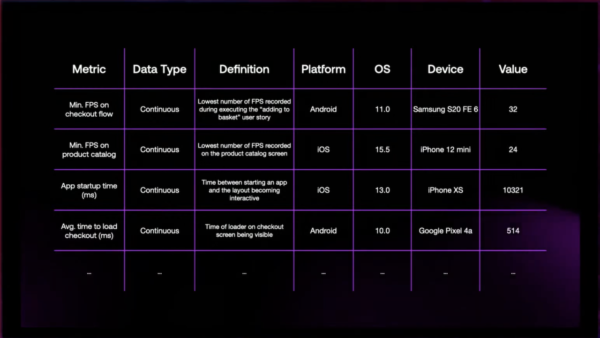
Measureme
- Measurement needs to be accurate and precise
Analyze phase: how do we get there?
- List potential root causes
- Cause and effect diagram (fish bone diagram)
- Narrow the list
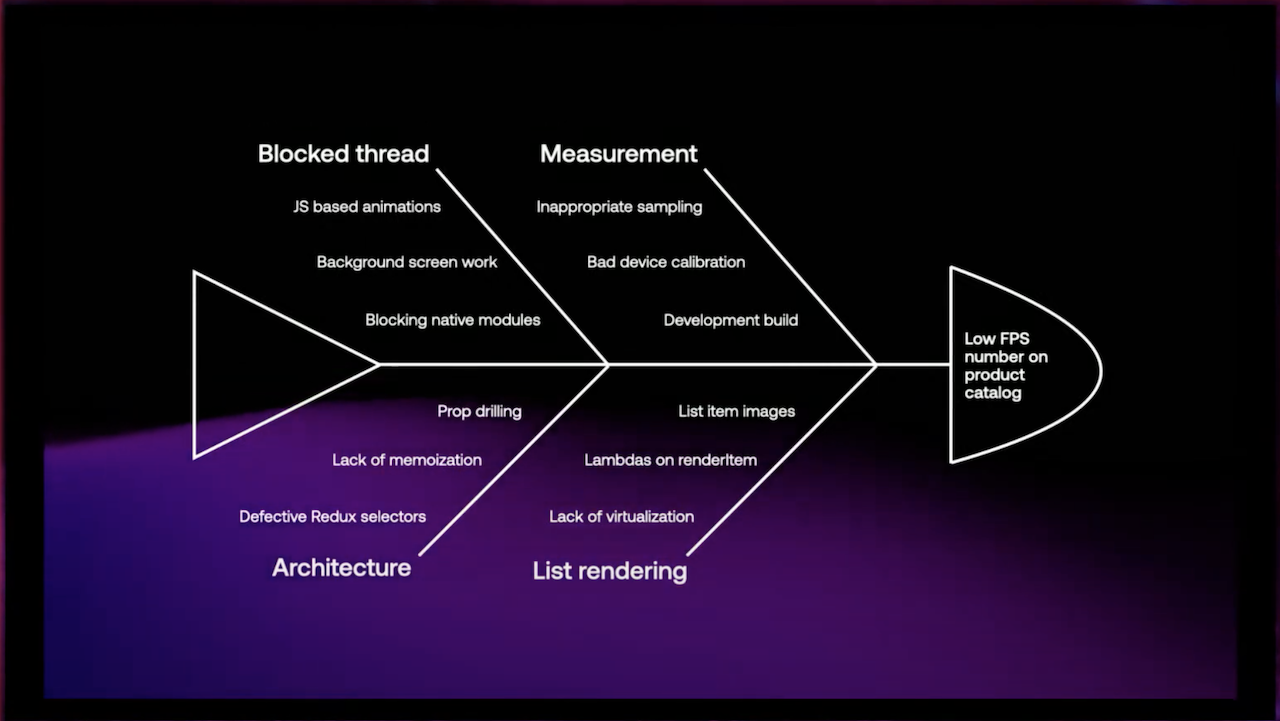
Improve phase
- Identify potential solutions
- Select the best solution
- Implement and test the solution
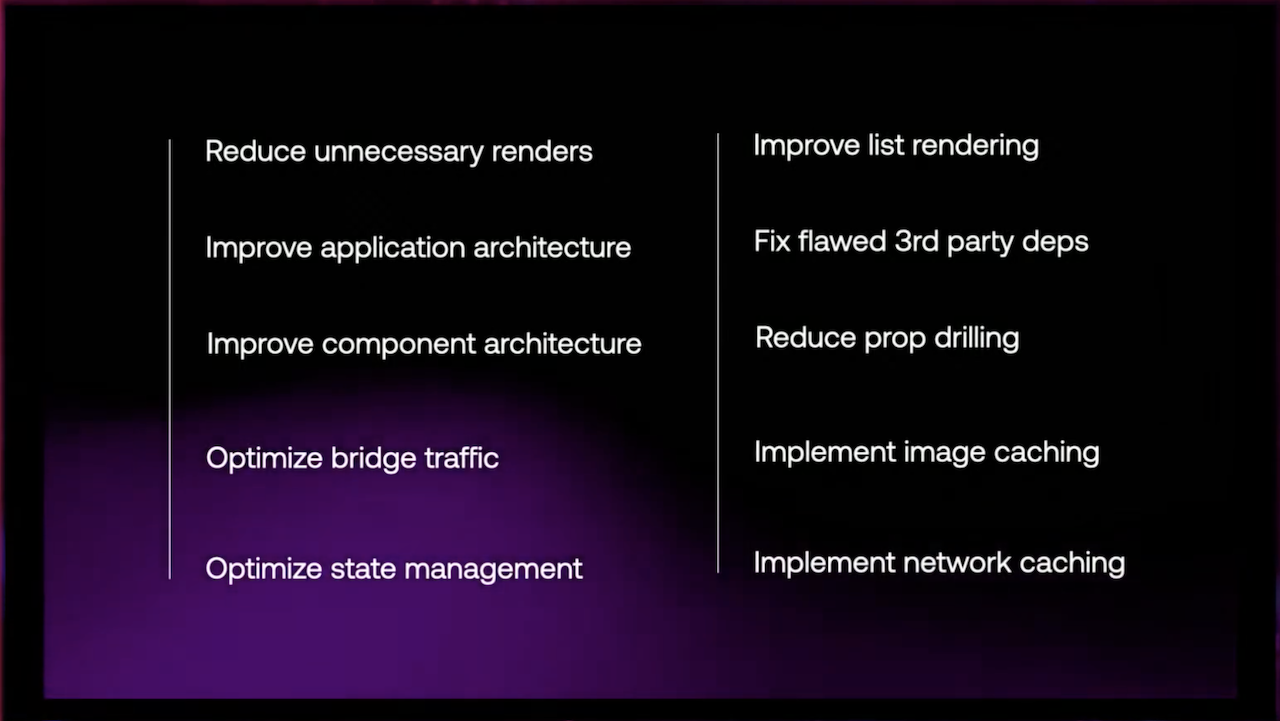
Control phase: are we on track?
Performance will degrade if it’s not under control so create a control plan.
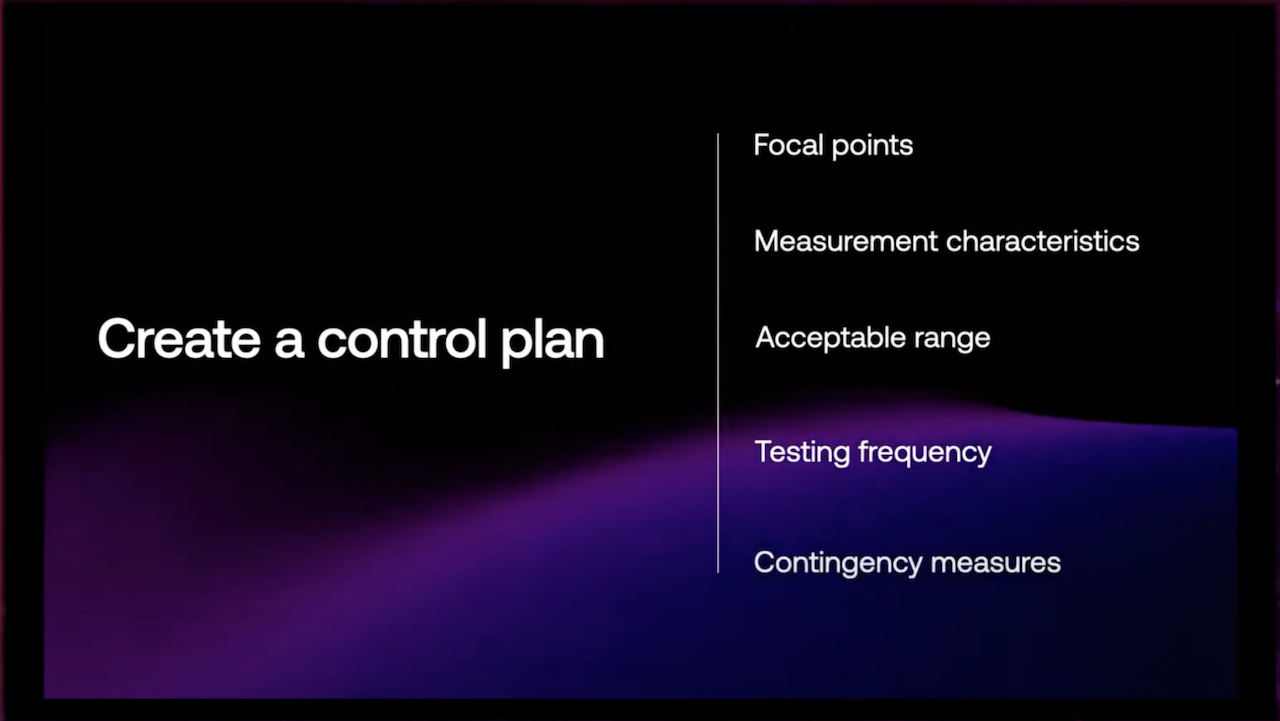
Monitor regressions
- Reassure: performance regression testing (local + CI)
- Firebase: performance monitoring on production
- Sentry: performance monitoring on production
Leave a Reply