We all write bug free code but analyzing your code is still important part of software development if for some reason there could’ve been some mishap with typing. Here’s a short introduction how to run static analysis for PHP code.
Static analysis tools for PHP
The curated list of static analysis tools for PHP show you many options for doing analysis. Too much you say? Yes but fortunately you can start with some tools and continue with the specific needs you have.
You can run different analysis tools by installing them with composer or you can use the Toolbox which helps to discover and install tools. You can use it as a Docker container.
First, fetch the docker image with static analysis tools for PHP:
$ docker pull jakzal/phpqa:<your php version>
e.g.
$ docker pull jakzal/phpqa:php7.4-alpine
PHPMD: PHP Mess Detector
One of the tools provided in the image is PHPMD which aims to be a PHP equivalent of the well known Java tool PMD. PHPMD can be seen as an user friendly and easy to configure frontend for the raw metrics measured by PHP Depend.
It looks for several potential problems within that source like:
- Possible bugs
- Suboptimal code
- Overcomplicated expressions
- Unused parameters, methods, properties
You can install the phpmd with composer: composer require phpmd/phpmd
. Then run it with e.g. ./vendor/bin/phpmd src html unusedcode --reportfile phpmd.html
Or run the command below which runs phpmd in a docker container and mounts the current working directory as a /project.
docker run -it --rm -v $(pwd):/project -w /project jakzal/phpqa:php7.4-alpine \
phpmd src html cleancode,codesize,controversial,design,naming,unusedcode --reportfile phpmd.html
You can also make your custom rules to reduce false positives: phpmd.test.xml
<?xml version="1.0"?> <ruleset name="VV PHPMD rule set" xmlns="http://pmd.sf.net/ruleset/1.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://pmd.sf.net/ruleset/1.0.0 http://pmd.sf.net/ruleset_xml_schema.xsd" xsi:noNamespaceSchemaLocation=" http://pmd.sf.net/ruleset_xml_schema.xsd"> <description> Custom rule set that checks my code. </description> <rule ref="rulesets/codesize.xml"> <exclude name="CyclomaticComplexity"/> <exclude name="ExcessiveMethodLength"/> <exclude name="NPathComplexity"/> <exclude name="TooManyMethods"/> <exclude name="ExcessiveClassComplexity"/> <exclude name="ExcessivePublicCount"/> <exclude name="TooManyPublicMethods"/> <exclude name="TooManyFields"/> </rule> <rule ref="rulesets/codesize.xml/TooManyFields"> <properties> <property name="maxfields" value="21"/> </properties> </rule> <rule ref="rulesets/cleancode.xml"> <exclude name="StaticAccess"/> <exclude name="ElseExpression"/> <exclude name="MissingImport" /> </rule> <rule ref="rulesets/controversial.xml"> <exclude name="CamelCaseParameterName" /> <exclude name="CamelCaseVariableName" /> <exclude name="Superglobals" /> </rule> <rule ref="rulesets/design.xml"> <exclude name="CouplingBetweenObjects" /> <exclude name="NumberOfChildren" /> </rule> <rule ref="rulesets/design.xml/NumberOfChildren"> <properties> <property name="minimum" value="20"/> </properties> </rule> <rule ref="rulesets/naming.xml"> <exclude name="ShortVariable"/> <exclude name="LongVariable"/> </rule> <rule ref="rulesets/unusedcode.xml"> <exclude name="UnusedFormalParameter"/> </rule> <rule ref="rulesets/codesize.xml/ExcessiveClassLength"> <properties> <property name="minimum" value="1500"/> </properties> </rule> </ruleset>
Then run your analysis with:
docker run -it --rm -v $(pwd):/project -w /project jakzal/phpqa:php7.4-alpine phpmd src html phpmd.test.xml unusedcode --reportfile phpmd.html
You get a list of found issues formatted to a HTML file
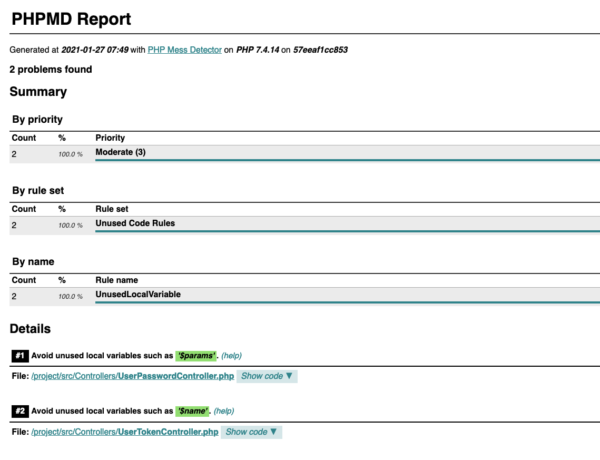
PHPStan – PHP Static Analysis Tool
“PHPstan focuses on finding errors in your code without actually running it. It catches whole classes of bugs even before you write tests for the code. It moves PHP closer to compiled languages in the sense that the correctness of each line of the code can be checked before you run the actual line.”
Installing with composer: composer require --dev phpstan/phpstan
Or run on Docker container:
docker run -it --rm -v $(pwd):/project -w /project jakzal/phpqa:php7.4-alpine phpstan analyse --level 1 src
By default you will get a report to console formatted to a table and grouped errors by file, colorized. For human consumption.
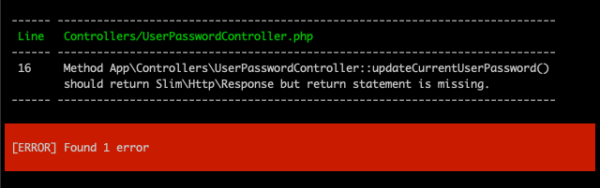
By default PHPStan is performing only the most basic checks and you can pass a higher rule level through the --level
option (0 is the loosest and 8 is the strictest) to analyse code more thoroughly. Start with 0 and increase the level as you go fixing possible issues.
PHPStan found some more issues which PHPMD didn’t find but the output of the PHPStan could be better. There’s a Web UI for browsing found errors and you can click and open your editor of choice on the offending line but you’ve to pay for it. PHPStan Pro costs 7 EUR for individuals monthly, 70 EUR for teams.
VS Code extension for PHP
If you’re using Visual Studio Code for PHP programming there are some extensions to help you.
PHP Intelephense
PHP code intelligence for Visual Studio Code provides better intellisense then VS Code builtin and also does some signature checking etc. The extension has also premium version for some additional features.
Leave a Reply