Software evolves quickly and new versions of libraries are released but how do you keep track of updated dependencies and vulnerable libraries? Managing dependencies has always been somewhat a pain point but an important part of software development as it’s better to be tracking vulnerabilities and running fresh packages than being pwned.
There are couple of tools for JavaScript projects which use npm to manage dependencies to check new versions and some tools to track vulnerabilities. Here’s a short introduction to npm audit, depcheck, npm-check-updates and npm-check to help you on your way.
If your project is using yarn adjust your workflow accordingly. There’s for example yarn audit and yarn-check to match tools for npm. And it goes without saying that don’t use npm if your project uses yarn.
Running security audit with npm audit
From version 6 onwards npm comes build with audit command which checks for vulnerabilities in your dependencies and runs automatically when you install a package with npm install. You can also run npm audit
manually on your locally installed packages to conduct a security audit of the package and produce a report of dependency vulnerabilities and suggested patches.
The npm audit command submits a description of the dependencies configured in your package to your default registry and asks for a report of known vulnerabilities. It checks direct dependencies, devDependencies, bundledDependencies, and optionalDependencies, but does not check peerDependencies.
If your npm registry doesn’t support npm audit, like Artifactory, you can pass in the --registry
flag to point to public npm. The downside is that now you can’t audit private packages that are on the Artifactory registry.
$ npm audit --registry=https://registry.npmjs.org
“Running npm audit
will produce a report of security vulnerabilities with the affected package name, vulnerability severity and description, path, and other information, and, if available, commands to apply patches to resolve vulnerabilities.”
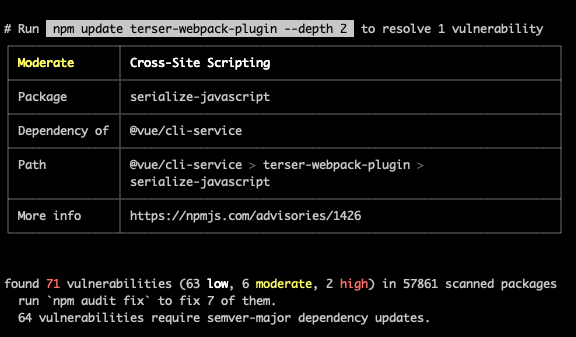
Using npm audit is useful also in Continuous Integration as it will return a non-zero response code if security vulnerabilities are found.
For more information read npm’s Auditing dependencies for security vulnerabilities.
Updating packages with npm outdated
It’s recommended to regularly update the local packages your project depends on to improve your code as improvements to its dependencies are made. In your project root directory, run the update command and then outdated. There should not be any output.
$ npm update $ npm outdated
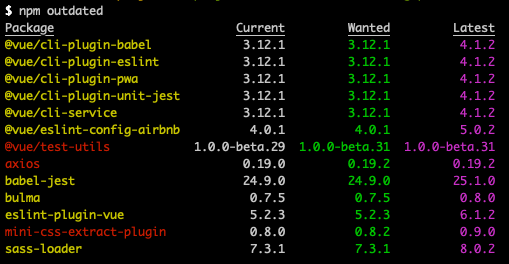
You can also update globally-installed packages. To see which global packages need to be updated run outdated first with –depth=0.
$ npm outdated -g --depth=0 $ npm outdated -g
For more information read updating packages downloaded from the registry.
Check updates with npm-check-updates
Package.json contains dependencies with semantic versioning policy and to find newer versions of package dependencies than what your package.json allows you need tools like npm-check-updates. It can upgrade your package.json dependencies to the latest versions, ignoring specified versions while maintaining your existing semantic versioning policies.
Install npm-check-updates globally with:
$ npm install -g npm-check-updates
And run it with:
$ ncu
The result shows any new dependencies for the project in the current directory. See documentation for i.a. configuration files for filtering and excluding dependencies.
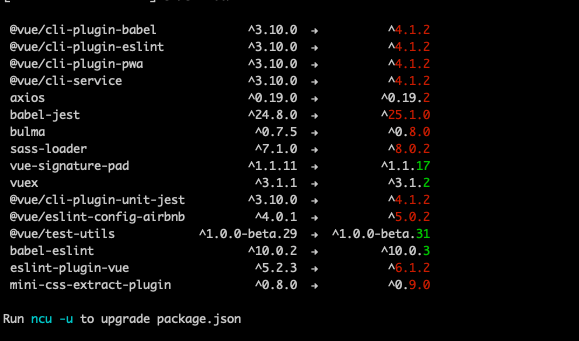
And finally you can run ncu -u
to upgrade the package.json.
Check updates with npm-check
Similar tool to npm-check-updates is npm-check which additionally gives more information about the version changes available and also lets you interactively pick which packages to update instead of an all or nothing approach. It checks for outdated, incorrect, and unused dependencies.
Install npm-check globally with:
$ npm i -g npm-check
Now you can run the command inside your project directory:
$ npm-check Or $ npm-check --registry=https://registry.npmjs.org
It will display all possible updates with information about the type of update, project URL, commands, and will attempt to check if the package is still in use. You can easily parse through the results and see what packages might be safe to update. When updates are required it will return a non-zero response code that you can use in your CI tools.
The check for unused dependencies uses depcheck and isn’t able to foresee all ways dependencies can be used so be vary with careless removing of packages.
To see an interactive UI for choosing which modules to update run:
$ npm-check –u
Analyze dependencies with depcheck
Your package.json is filled with dependencies and some of them might be useless or even missing from package.json. Depcheck is a tool for analyzing the dependencies in a project to see how each dependency is used, which dependencies are useless, and which dependencies are missing. It does not only recognizes the dependencies in JavaScript files, but also supports i.a. React JSX and Typescript.
Install depcheck with:
$ npm install -g depcheck And with additional syntax support for Typescript $ npm install -g depcheck typescript
Run depcheck with:
$ depcheck [directory]
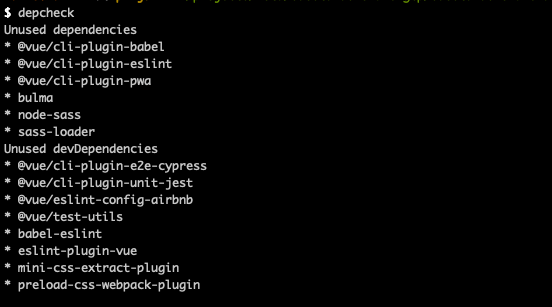
Summary
tl;dr;
- Use
npm audit
in your CI pipeline - Update dependencies with
npm outdated
- Check new versions of dependencies with either
npm-check-updates
ornpm-check
- Analyze dependencies with
depcheck
Leave a Reply